Angular Js
Introduction
This article is about CRUD Operation in SharePoint 2013/2010 using Angular Js.
Let's Start
Let's complete this task in following steps:-
- Create a simple web part page. I have created the same, in my Online SharePoint Site named it as EmpSysDetails.aspx.
- Create a custom list with the columns, which will store values of user input.
- Create an AngularJs folder in Site Assets library. Upload Angular.js file. You can download it from following site https://angularjs.org/ .
- Mention uploaded js script link in html markup file or you can use CDN link.
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.1/angular.js"></script>
- Create html mark-up with ng-app and ng-controller directive.
<div ng-app="myApp" ng-controller="myCtrl">
- Create a Table using ng-repeat directive.
<table class="table" style="margin-left:10px;">
<tr>
<th>
Employee Name
</th>
<th>
Employee Dept
</th>
<th>
Desk No
</th>
<th>
System Alloted
</th>
<th>Edit</th>
<th>Delete</th>
</tr>
<tr ng-repeat="x in employees">
<td>{{x.EmpName}}</td>
<td>{{x.EmpDept}}</td>
<td>{{x.EmpDeskNo}}</td>
<td ng-if="x.SysAllocated">Yes</td>
<td ng-if="! x.SysAllocated">No</td>
<td><img ng-click="EditItem(x.ID)" ng-src="../SiteAssets/AngularJs/Images/edit.png" width="50px" height="50px" alt="Edit"></img></td>
<td><img ng-click="DeleteItem(x.ID)" ng-src="../SiteAssets/AngularJs/Images/delete-512.png" width="50px" height="50px" alt="Delete"></img></td>
</tr>
</table>
- Create Form Elements,for instance text box and checkbox using ng-model directive.
<div class="container">
<div class="row">
<div class="col-md-8 setrow">
<span class="col-md-4">Title</span> <input class="form-control" type="text" ng-model="title" placeholder="Title" />
</div>
</div>
<div class="row">
<div class="col-md-8 setrow">
<span class="col-md-4">Employee Name</span> <input type="text" class="form-control" ng-model="EmpName" placeholder="Enter Employee Full Name" />
</div>
</div>
</div>
- Include appropriate CSS using Bootstarp.css and custom css.
- Create two separate JavaScript File, name it as appscript.js and sharepointfactory.js.
- appScript.js file contains angular app module and controller code.
- In Controller code inject "$scope" object and "SharePointFactory" service (Name of angular factory).
var
app = angular.module("myApp",[]); app.controller("myCtrl",
function
($scope,SharePointFactory){ });
- sharepointfactory.js file contains REST API call to do CRUD operation.
- In the mentioned angular factory inject $http and $q service.
app.factory('SharePointFactory', ['$http','$q',
function
($http,$q) { }]);
- Upload these two files in Site Assets library's AngulaJs folder. Mention script link in the html markup file.
<script src="../SiteAssets/AngularJs/appscript.js"></script>
<script src="../SiteAssets/AngularJs/sharepointfactory.js"></script>
- Edit the EmpSysDetails.aspx. page and add a Script editor webpart.
- Click on Edit Snippet.Copy and paste all the html markup including script link into the editor window.
- Click on Save button present in top bar, ribbon.
EmpSysDetails.aspx looks like as shown below:-
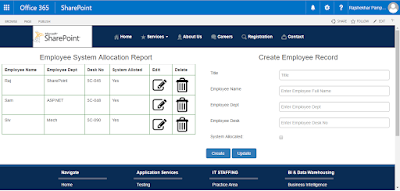
Demystifying sharepointfactory.js file.
Let's dive into REST call to do CRUD operation.All the REST call has been done using $http and
$q service in angular factory written in sharepointfactory.js file.
* Fetch list items from SharePoint list i.e EmployeeList
var
getData =
function
() {
return
$http.get(_spPageContextInfo.siteAbsoluteUrl+"/Proctor/_api/Web/Lists/GetByTitle('EmployeeList')/items?select=ID,Title,EmpName,EmpDept,EmpDeskNo,SysAllocated") .
then
(
function
(response) {
return
response.data.value; }); }
* Create a list item in EmployeeList
var
crtItem =
function
(data) {
var
deferred = $q.defer(); $http({ url: _spPageContextInfo.siteAbsoluteUrl+"/Proctor/_api/Web/Lists/GetByTitle('EmployeeList')/items", method: "POST", headers: { "accept": "application/json;odata=verbose", "X-RequestDigest": $("#__REQUESTDIGEST").val(), "content-Type": "application/json;odata=verbose" }, data: JSON.stringify(data) }).then(
function
success(response){ deferred.resolve(response); },
function
error(result,status){ deferred.reject(status); });
return
deferred.promise; }
* Fetch specific list item from EmployeeList by Id
var
edtItem =
function
(Id) {
return
$http.get(_spPageContextInfo.siteAbsoluteUrl+"/Proctor/_api/Web/Lists/GetByTitle('EmployeeList')/GetItemById(" + Id + ")") .then(
function
(response) {
return
response.data; }); }
* Update specific list item
var
updtitem =
function
(data){ console.log(data.ID);
var
deferred = $q.defer(); $http({ url: _spPageContextInfo.siteAbsoluteUrl+"/proctor/_api/web/lists/getbytitle('employeelist')/getitembyid(" + data.ID + ")", method: "PATCH", headers: { "accept": "application/json;odata=verbose", "X-RequestDigest": $("#__REQUESTDIGEST").val(), "content-type": "application/json;odata=verbose", "X-HTTP-Method": "PATCH", 'IF-MATCH': '*' }, data: JSON.stringify(data) }).then(
function
success(response){ deferred.resolve(response); },
function
error(result,status){ deferred.reject(status); });
return
deferred.promise; }
* Delete specific list item by Id
var
dltItem =
function
(Id) {
var
deferred = $q.defer(); $http({ url: _spPageContextInfo.siteAbsoluteUrl+"/Proctor/_api/Web/Lists/GetByTitle('EmployeeList')/items(" + Id + ")", method: "DELETE", headers: { "accept": "application/json;odata=verbose", "X-RequestDigest": $("#__REQUESTDIGEST").val(), 'IF-MATCH': '*' }, }).then(
function
success(response){ deferred.resolve(response); },
function
error(result,status){ deferred.reject(status); });
return
deferred.promise; }
*Mention return object in angular factory.
return
{ getData: getData , crtItem : crtItem, edtItem : edtItem, dltItem :dltItem, updtitem:updtitem };
Demystifying appScript.js file.
- $scope.employees declared as an array object. getData() method from "SharepointFactory" service to fetch list item and update array object. It will create table row with content in it.
var
app = angular.module("myApp",[]); app.controller("myCtrl",
function
($scope,SharePointFactory){ $scope.employees = [];
// Get all the items from the SharePoint List
SharePointFactory.getData().then(
function
(emp){ angular.forEach(emp,
function
(item,index){ $scope.employees.push(item); }); });
2. The event.preventDefault() method stops the default action of an element from happening. For example: Prevent a submit button from submitting a form.
$scope.createItem() event trigger on click of Create Button. It creates an item in list.and updates array object.The powerful feature of angular js - Two-way binding, creates a new row and content in the table.
// Create an Item in SharePoint List
$scope.createItem =
function
(event){ event.preventDefault();
var
data = { __metadata: { 'type': 'SP.Data.EmployeeListListItem' }, Title:$scope.title, EmpName:$scope.EmpName, EmpDept:$scope.EmpDept, EmpDeskNo:$scope.EmpDesk, SysAllocated:$scope.SysAllocated }; SharePointFactory.crtItem(data).then(
function
(response) { $scope.employees.push(response.data.d); $scope.title = ""; $scope.EmpName = ""; $scope.EmpDesk= ""; $scope.EmpDept= ""; $scope.SysAllocated = false; }); };
// Edit an Item in SharePoint List
$scope.EditItem = function(ID){ SharePointFactory.edtItem(ID).then(
function
(emp){ $scope.title = emp.Title; $scope.EmpName = emp.EmpName; $scope.EmpDept = emp.EmpDept; $scope.EmpDesk = emp.EmpDeskNo; $scope.SysAllocated = emp.SysAllocated; $scope.ID = emp.ID; }); };
4. $scope.updateItem() event trigger on click of Update button. It will update the edited item and the splice() method adds/removes items to/from an array. It will update the UI with updated content in table row.
// Update an item in Sharepoint List
$scope.updateItem =
function
(event){ event.preventDefault(); var data = { __metadata: { 'type': 'SP.Data.EmployeeListListItem' }, Title:$scope.title, EmpName:$scope.EmpName, EmpDept:$scope.EmpDept, EmpDeskNo:$scope.EmpDesk, SysAllocated:$scope.SysAllocated, ID:$scope.ID }; SharePointFactory.updtitem(data).then(
function
(response) { angular.forEach($scope.employees,
function
(item,index){ if(item.ID == data.ID){ $scope.employees.splice(index,1,data); $scope.title = ""; $scope.EmpName = ""; $scope.EmpDesk= ""; $scope.EmpDept= ""; $scope.SysAllocated = false; } }); }); };
5. $scope.DeleteItem() event trigger on click of Delete Icon in the Table. It will delete the list item and remove that item from the array object.It will remove the row from the table.
// Delete an Item in SharePoint List
$scope.DeleteItem =
function
(ID){ SharePointFactory.dltItem(ID).then(
function
(emp){ $scope.removeItem($scope.employees,ID); }); }; $scope.removeItem =
function
(array, ID){ console.log("remove item"); angular.forEach(array,
function
(item,index){ if(item.ID == ID){ $scope.employees.splice(index,1); $scope.title = ""; $scope.EmpName = ""; $scope.EmpDesk= ""; $scope.EmpDept= ""; $scope.SysAllocated = false; } }); };